Android Application Development
Introduction:
Android application development is the process of creating mobile applications for Android operating systems using the Android SDK. The Android operating system is the most widely used mobile operating system in the world, making it an essential skill for mobile app developers.
Learning Android Application Development:
There are many reasons why learning Android application development is important. The most significant of these is the growing demand for mobile applications. As the use of mobile devices continues to grow, there is an increased demand for developers who can create innovative and engaging applications for Android devices.
Getting Started with Android App Development
Android Studio IDE is the primary tool used for developing Android applications. Android Studio is an Integrated Development Environment (IDE) that provides a comprehensive set of tools for developing, testing, and debugging Android applications. In this chapter, we will discuss how to install and configure Android Studio, the basic components of an Android app, such as activities, fragments, layouts, and views, and provide a simple example of an Android app.
Creating User Interface
The user interface (UI) is an essential aspect of any mobile application. It is what the user interacts with, and it must be easy to use, visually appealing, and responsive. In this chapter, we will discuss the different types of layouts available in Android, such as Linear Layout, Relative Layout, and Constraint Layout. We will also explain how to create UI elements such as buttons, text fields, and images, and demonstrate how to apply styles and themes to your app.
Handling User Input
User input is an essential part of any mobile application. In this chapter, we will explain how to capture user input through different UI elements such as buttons, text fields, and check boxes. We will also discuss how to validate user input and display error messages and demonstrate how to use the Input Method Manager to show and hide the on-screen keyboard.
Working with Data
Data storage is a crucial component of most mobile applications. In this chapter, we will discuss how to store data in Shared Preferences, introduce the SQLite database, and demonstrate how to create, read, update, and delete data from a database. We will also explain how to use Content Providers to share data between apps.
Developing Android applications requires a thorough understanding of the Android SDK, Android Studio, and related technologies. This guide has provided an overview of the basics of Android application development, including how to get started with Android Studio, creating user interfaces, handling user input, and working with data. By following best practices and staying up-to-date with the latest developments in Android app development, developers can create innovative and engaging applications for Android devices.
Networking and Web Services
Networking and web services are essential components of modern mobile app development. In this chapter, we will introduce HTTP and RESTful web services and discuss how to make HTTP requests using libraries such as Retrofit and Volley. We will also explain how to parse JSON and XML data using Gson and XmlPullParser.
HTTP and RESTful web services are widely used in Android app development to enable communication between the app and a web server. RESTful web services use HTTP protocols to create web APIs that can be used to request and receive data from a server. In order to interact with RESTful web services, you need to understand HTTP methods such as GET, POST, PUT, and DELETE.
To make HTTP requests in Android, you can use libraries such as Retrofit and Volley. Retrofit is a popular HTTP client library that allows you to easily send HTTP requests and handle responses in your Android app. Volley is another HTTP library that provides support for caching, request prioritization, and network queuing.
JSON and XML are the two most common data interchange formats used in web services. In Android, you can parse JSON and XML data using libraries such as Gson and XmlPullParser. Gson is a Java library that converts Java Objects into their JSON representation and vice versa. XmlPullParser is a lightweight and efficient XML parsing library that can be used to parse XML data in Android apps.
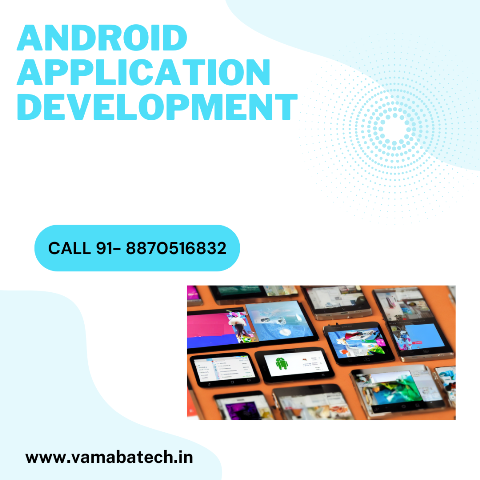
Working with Multimedia
Multimedia features such as audio, video, and images are common in modern Android apps. In this chapter, we will discuss how to play audio and video files in your app using the MediaPlayer and ExoPlayer libraries. We will also explain how to capture photos and videos using the Camera2 API.
The MediaPlayer library is a built-in Android class that provides playback support for audio and video files. ExoPlayer is an advanced media player library that provides support for features such as adaptive streaming, video caching, and live streaming. You can use these libraries to create multimedia-rich apps that provide a great user experience.
The Camera2 API is a powerful and flexible camera framework that allows you to access and control camera features on Android devices. With this API, you can capture photos and videos, apply effects, and control camera settings such as focus, exposure, and white balance.
Advanced Topics
Android app development offers a wide range of advanced topics that can help you create powerful and efficient apps. In this chapter, we will discuss how to use services and broadcast receivers to run background tasks. We will also introduce Android Jetpack, including ViewModel, LiveData, and Room. Finally, we will explain how to use fragments for better app organization and navigation.
Services are a key feature of Android that allows you to run tasks in the background even when the app is not in use. Broadcast receivers are another Android component that enables your app to respond to system events such as incoming calls or text messages. By using these components, you can create apps that provide a seamless user experience and work efficiently in the background.
Android Jetpack is a set of libraries, tools, and architectural guidance provided by Google to help you build high-quality Android apps. ViewModel is a Jetpack library that provides a way to manage and store UI-related data in a lifecycle-aware manner. LiveData is another Jetpack library that provides observable data objects that can be used to update the UI automatically. Room is a Jetpack library that provides an abstraction layer over SQLite database and simplifies database operations.
Fragments are UI components in Android that represent a portion of a user interface. They are used to build flexible UI layouts for different screen sizes and orientations. By using fragments, you can create apps that are optimized for both phones and tablets.
Types of Android Applications
There are several distinct categories into which Android applications can be broadly classified :
Native Apps
These are applications that are specifically developed for the Android platform using programming languages such as Java or Kotlin. These apps offer the best performance and are optimized for the Android platform.
Hybrid Apps
Hybrid apps refer to mobile applications that blend aspects of both native and web apps. Developers construct them using HTML, CSS, and JavaScript, which are common web technologies, and then wrap them in a native container.They are less expensive to develop than native apps and can be deployed on multiple platforms.
Web Apps
These are mobile versions of websites that are optimized for viewing on mobile devices. They are accessed through a web browser and do not require any installation.
Benefits of Android Application Development
Android application development offers numerous benefits, including:
Large User Base
Android has the largest user base of any mobile operating system, with over 2 billion active users. Developing apps for Android means having access to a large and growing market.
Open-Source Platform
Android is an open-source platform, which means that developers can modify the code to suit their needs. The flexibility and customization of software applications can be enhanced through a variety of methods.
Cost-Effective
Developing Android apps can be more cost-effective than developing apps for other platforms, as the Android SDK is free and there are fewer restrictions on app development.
Design Considerations for Android Applications
When designing Android applications, it is important to consider the following factors:
User Interface
The user interface should be intuitive and easy to use, with clear navigation and appropriate use of color and typography.
Device Compatibility
Android applications should be designed to work across a range of devices with varying screen sizes and resolutions.
Performance
The app should be optimized for performance, with efficient code and appropriate use of resources.
Android App Development Tools and Platforms
There are several tools and platforms available for Android app development, including:
Android Studio
Android app developers typically rely on a designated integrated development environment (IDE) for their development needs. It offers a range of features, including code editing, debugging, and performance analysis.
Xamarin
This is a cross-platform development tool that allows developers to create Android apps using C#.
React Native
This is a popular cross-platform development tool that allows developers to create Android apps using JavaScript.
Android application development offers numerous benefits and requires careful consideration of design and development tools. By understanding the various types of Android applications, developers can choose the most appropriate development approach for their needs.
Steps for Android App Development Process
The development process for Android applications can vary depending on the project and its requirements, but there are some common steps that can be followed. Here are the typical steps in the Android app development process:
Idea and Conceptualization
This is where the app idea is generated and the purpose of the app is defined.
Planning
This step involves defining the features of the app, creating a project plan, and estimating the resources required.
Design
This stage focuses on creating the user interface, deciding on the app’s layout, and making sure the design is user-friendly.
Development
This is where the coding of the app takes place. This includes building the user interface, adding functionality to the app, and integrating backend services.
Testing
This step involves checking the app for bugs, defects, and issues. The app is tested to ensure that it functions correctly and is user-friendly.
Deployment
After testing is complete and the app is ready, it can be published to app stores for download.
Android App Testing and Quality Assurance
The process of testing is integral to app development. It helps to ensure that the app is working correctly and meets the user’s requirements. Quality assurance (QA) involves a set of activities that help to ensure that the app is of high quality. Here are some testing and quality assurance practices for Android app development:
Manual Testing
This is the process of manually testing the app to ensure that it is working correctly. Manual testing is often performed by testers who use the app to find defects, issues, and bugs.
Automated Testing
This involves using automated tools and scripts to test the app. Automated testing helps to reduce the time and effort required for testing.
User Acceptance Testing
This involves getting feedback from users on the app’s functionality, usability, and design.
Performance Testing
This is the process of testing the app’s performance under different conditions, such as low battery, low memory, and poor network conditions.
Security Testing
This involves testing the app’s security features, such as authentication, encryption, and data protection.
Best Practices for Developing Android Applications
Here are some best practices for developing Android applications:
Follow the Material Design Guidelines
The Material Design Guidelines provide a set of design principles that help to create user-friendly and visually appealing apps.
Optimize the App’s Performance
The app’s performance is crucial for user satisfaction. Therefore, it’s essential to optimize the app’s performance by reducing load times, minimizing memory usage, and improving battery life.
Use Clean and Maintainable Code
Clean and maintainable code is easy to understand and modify, which makes it easier to maintain and update the app.
Follow the Principles of Separation of Concerns
Separation of concerns is a programming principle that involves separating different parts of the app into separate modules, such as user interface, business logic, and data storage.
Keep Security in Mind
Security should be a top priority when developing an app. It’s essential to use secure coding practices and implement security features such as encryption and data protection.
Test the App Thoroughly
Testing is crucial to ensure that the app is functioning correctly and is user-friendly. It’s essential to test the app thoroughly using manual and automated testing techniques.
Keep the User Experience in Mind
The success of an app is heavily dependent on providing an exceptional user experience. Therefore, it’s essential to keep the user experience in mind when designing and developing the app.
In conclusion, Android application development is a complex but rewarding process. By learning how to create Android apps, you can build powerful, feature-rich applications that can run on millions of devices worldwide.
Throughout this blog, we have covered the key components of Android application development, including getting started with Android Studio, creating user interfaces, handling user input, working with data, networking and web services, working with multimedia, and advanced topics such as services, broadcast receivers, and Android Jetpack.
We have also discussed the different types of Android applications and the benefits of developing them, as well as design considerations, development tools, and best practices to ensure high-quality app development.
However, the development process doesn’t end with writing code. Testing and quality assurance are critical steps in the process, ensuring that your app works as intended and provides a good user experience. By following best practices and using testing tools such as JUnit, Espresso, and Firebase Test Lab, you can catch bugs and issues before your app is released to the public.
In summary, Android application development requires a combination of technical skills, creativity, and attention to detail. With the right tools and knowledge, you can create apps that users love and that make a positive impact in the world. So keep learning, experimenting, and pushing the boundaries of what’s possible with Android app development.